In this tutorial we will show how to build ESP8266 and interface DS18B20 temperature sensor and LCD display. This project uses ESP8266 NodeMCU device that easily connects to existing WiFi network & creates a Web Server. When any connected device accesses this web server, ESP8266 reads in temperature from DS18B20 Temperature sensors & sends it to the web browser.
Circuit diagram

Components Required
ESP8266 12E
DS18B20 with Probe
LCD 16X2 with I2C
Jumper wire
DS18B20

The DS18B20 is a 1-wire programmable Temperature sensor from maxim integrated. It is widely used to measure temperature in hard environments like in chemical solutions, mines or soil etc. The constriction of the sensor is rugged and also can be purchased with a waterproof option making the mounting process easy. It can measure a wide range of temperature from -55°C to +125° with a decent accuracy of ±5°C. Each sensor has a unique address and requires only one pin of the MCU to transfer data so it a very good choice for measuring temperature at multiple points without compromising much of your digital pins on the microcontroller.
How to use the DS18B20 Sensor
The sensor works with the method of 1-Wire communication. It requires only the data pin connected to the microcontroller with a pull up resistor and the other two pins are used for power as shown below.

The pull-up resistor is used to keep the line in high state when the bus is not in use. The temperature value measured by the sensor will be stored in a 2-byte register inside the sensor. This data can be read by the using the 1- wire method by sending in a sequence of data. There are two types of commands that are to be sent to read the values, one is a ROM command and the other is function command. The address value of each ROM memory along with the sequence is given in the datasheet below. You have to read through it to understand how to communicate with the sensor.
If you are planning to interface it with Arduino, then you need not worry about all these. You can develop the readily available library and use the in-built functions to access the data.
Applications
Measuring temperature at hard environments
Liquid temperature measurement
Applications where temperature has to be measured at multiple points
DS18B20 Sensor Specifications
Programmable Digital Temperature Sensor
Communicates using 1-Wire method
Operating voltage: 3V to 5V
Temperature Range: -55°C to +125°C
Accuracy: ±0.5°C
Output Resolution: 9-bit to 12-bit (programmable)
Unique 64-bit address enables multiplexing
Conversion time: 750ms at 12-bit
Programmable alarm options
Available as To-92, SOP and even as a waterproof sensor
Installing the ESPAsyncWebServer library
The ESPAsyncWebServer library is not available to install in the Arduino IDE Library Manager. So, you need to install it manually.
Click here to download the https://github.com/me-no-dev/ESPAsyncWebServer/archive/master.zip
library. You should have a .zip folder in your Downloads folder Unzip the .zip folder and you should get ESPAsyncWebServer-master folder
Rename your folder from ESPAsyncWebServer-master to ESPAsyncWebServer
Move the ESPAsyncWebServer folder to your Arduino IDE installation libraries folder
Subscribe and Download code.
Installing Libraries for DS18B20
To interface with the DS18B20 temperature sensor, you need to install the One Wire library by Paul Stoffregen and the Dallas Temperature library. Follow the next steps to install those libraries.
1. Open your Arduino IDE and go to Sketch > Include Library > Manage Libraries. The Library Manager should open.
2. Type “onewire” in the search box and install the OneWire library by Paul Stoffregen.
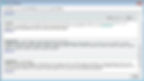
3. Then, search for “Dallas” and install the Dallas Temperature library by Miles Burton.
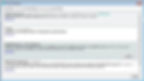
After installing the libraries, restart your Arduino IDE.
Subscribe and Download code.
Arduino Code
#ifdef ESP32
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <Hash.h>
#include <ESPAsyncTCP.h>
#include <ESPAsyncWebServer.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27,16,2);
// Data wire is connected to GPIO 4
#define ONE_WIRE_BUS 2
// Setup a oneWire instance to communicate with any OneWire devices
OneWire oneWire(ONE_WIRE_BUS);
// Pass our oneWire reference to Dallas Temperature sensor
DallasTemperature sensors(&oneWire);
// Replace with your network credentials
const char* ssid = "TP-Link_3200"; //YOUR WIFI ssid
const char* password = "9500112137"; //YOUR WIFI PASSWORD
// Create AsyncWebServer object on port 80
AsyncWebServer server(80);
String readDSTemperatureC() {
// Call sensors.requestTemperatures() to issue a global temperature and Requests to all devices on the bus
sensors.requestTemperatures();
float tempC = sensors.getTempCByIndex(0);
if(tempC == -127.00) {
Serial.println("Failed to read from DS18B20 sensor");
return "--";
} else {
Serial.print("Temperature Celsius: ");
Serial.println(tempC);
lcd.setCursor(0,0);
lcd.print(" Celsius: ");
lcd.setCursor(11,0);
lcd.print(tempC);
}
return String(tempC);
}
String readDSTemperatureF() {
// Call sensors.requestTemperatures() to issue a global temperature and Requests to all devices on the bus
sensors.requestTemperatures();
float tempF = sensors.getTempFByIndex(0);
if(int(tempF) == -196){
Serial.println("Failed to read from DS18B20 sensor");
return "--";
} else {
Serial.print("Temperature Fahrenheit: ");
Serial.println(tempF);
lcd.setCursor(0,1);
lcd.print("Fahrenheit:");
lcd.setCursor(11,1);
lcd.print(tempF);
}
return String(tempF);
}
const char index_html[] PROGMEM = R"rawliteral(
<!DOCTYPE HTML><html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.7.2/css/all.css" integrity="sha384-fnmOCqbTlWIlj8LyTjo7mOUStjsKC4pOpQbqyi7RrhN7udi9RwhKkMHpvLbHG9Sr" crossorigin="anonymous">
<style>
html {
font-family: Arial;
display: inline-block;
margin: 0px auto;
text-align: center;
}
h2 { font-size: 2.0rem; }
p { font-size: 2.0rem; }
.units { font-size: 1.2rem; }
.ds-labels{
font-size: 1.5rem;
vertical-align:middle;
padding-bottom: 15px;
}
</style>
</head>
<body>
<h2>NodeMCU Based DS18B20 WebServer</h2>
<p>
<i class="fas fa-thermometer" style="color:#07f0d1;"></i>
<span class="ds-labels">Temperature</span>
<span id="temperaturec">%TEMPERATUREC%</span>
<sup class="units">°Celsius</sup>
</p>
<p>
<i class="fas fa-thermometer" style="color:#07f0d1;"></i>
<span class="ds-labels">Temperature</span>
<span id="temperaturef">%TEMPERATUREF%</span>
<sup class="units">°Farenheit</sup>
</p>
</body>
<script>
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("temperaturec").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/temperaturec", true);
xhttp.send();
}, 10000) ;
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("temperaturef").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/temperaturef", true);
xhttp.send();
}, 10000) ;
</script>
</html>)rawliteral";
// Replaces placeholder with DHT values
String processor(const String& var){
//Serial.println(var);
if(var == "TEMPERATUREC"){
return readDSTemperatureC();
}
else if(var == "TEMPERATUREF"){
return readDSTemperatureF();
}
return String();
}
void setup(){
// Serial port for debugging purposes
Serial.begin(115200);
Serial.println();
lcd.init(); // initialize the lcd
// Print a message to the LCD.
lcd.backlight();
// Start up the DS18B20 library
sensors.begin();
// Connect to Wi-Fi
WiFi.begin(ssid, password);
Serial.println("Connecting to WiFi");
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println();
// Print ESP Local IP Address
Serial.println(WiFi.localIP());
// Route for root / web page
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/html", index_html, processor);
});
server.on("/temperaturec", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", readDSTemperatureC().c_str());
});
server.on("/temperaturef", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", readDSTemperatureF().c_str());
});
// Start server
server.begin();
}
void loop(){
}
Then, upload the code to your NodeMCU board. Make sure you have selected the right board and COM port. Also, make sure you’ve inserted your WiFi Credentials in the code.

After a successful upload, open the Serial Monitor at a baud rate of 115200. Press the “EN/RST” button on the ESP8266 board. Now it should print its IP address. After That Open web address and Type IP address in address bar and get result.