Learn the interfacing Metal Touch Sensor Module KY-036 in ESP8266 12E. The touch sensor will generate certain output on touching metal spike of the sensor. Measured input from the Sensor is then fed to the amplifier. Amplifier then sends analog / Digital data to the analog / Digital output pin of the module. In this, we are going to interface, LCD, HTTP server for Real time data logger, Buzzer using the Metal Touch Sensor Module KY-036 / KSP13 with NodeMCU esp8266 12E.
Circuit Diagram

Components required
nodeMCU ESP8266 12E
KY-036 Metal Touch Sensor
LCD 16x2 I2C
Buzzer
KY-036 Metal Touch sensor
KY-036 Metal touch sensor module Very simple – the touch sensor (KSP13-NPN Epitaxial Silicon Darlington Transistor) will generate an output when the metal leg of the KSP13 is touched. This is then fed to the LM386 amplifier. The amplifier then sends the data to the digital output pin of the module.
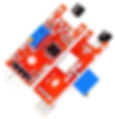
LED1: Shows that the sensor is supplied with voltage
LED2: Shows that the sensor detects a magnetic field
Outputs a signal if the metal pike of the Sensor was touched. You can adjust the sensitivity of the sensor with the controller.
Digital Out: At the moment of contact detection, a signal will be outputted.
Analog Out: Direct measuring value of the sensor unit.

The sensor has 3 main components on its circuit board. First, the sensor unit at the front of the module which measures the area physically and sends an analog signal to the second unit, the amplifier. The amplifier amplifies the signal, according to the resistant value of the potentiometer, and sends the signal to the analog output of the module.The third component is a comparator which switches the digital out and the LED if the signal falls under a specific value.
You can control the sensitivity by adjusting the potentiometer. The signal will be inverted; that means that if you measure a high value, it is shown as a low voltage value at the analog output.
Buzzer
Sounds when touched the Metal touch sensor.
Positive: Identified by (+) symbol or longer terminal lead. Can be Connected to NodeMCU pin D4.
Negative: Identified by short terminal lead. Typically connected to the ground of the circuit.
Rated Voltage: 6V DC
Operating Voltage: 4-8V DC
Rated current: <30mA
LCD 16x2 (I2C module)
This is a 16x2 LCD display screen with I2C interface. It is able to display 16x2 characters on 2 lines, white characters on blue background.
This I2C 16x2 Arduino LCD Screen is using an I2C communication interface. It means it only needs 4 pins for the LCD display: VCC, GND, SDA, SCL.
Connecting the LCD to NodeMCU
Connect the VCC I2C pin to a Vin pin on the NodeMCU.
Connect the GND I2C pin to a GND pin on the NodeMCU.
Connect the SCL I2C pin to a D1 pin on the NodeMCU.
Connect the SDA I2C pin to a D2 pin on the NodeMCU.
Installing Libraries
LiquidCrystal_I2C.h : you need to Download and install the LiquidCrystal_I2C library.
In your Arduino IDE, to install the libraries go to Sketch > Include Library > Add .ZIP library… and select the library you’ve just downloaded.
After installing the required libraries, copy the following code to your Arduino IDE.
Subscribe and Download code.
Subscribe and Download code.
Arduino Code
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266WebServer.h>
const char* ssid = "TP-Link_3200"; // your SSID
const char* password = "9500112137"; //your WIFI PASSWORD
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27,16,2);
ESP8266WebServer server(80); //Server on port 80
int buzzer = 2; //Buzzer alarm connected to GPIO-14 or D5 of nodemcu
int MetalTouch = 16; //Metal sensor output connected to GPIO-5 or D1 of nodemcu
String Message;
const char MAIN_page[] PROGMEM = R"=====(
<!doctype html>
<html>
<head>
<title>Data Logger</title>
<h1 style="text-align:center; color:#605034;">Iot Based Metal Touch Detector</h1>
<h3 style="text-align:center;color:#7f725c;">Real Time Data for Touch Log</h3>
<style>
canvas{
-moz-user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
}
/* Data Table Styling*/
font-family: Trebuchet MS, Arial, "Helvetica", sans-serif;
border-collapse: collapse;
width: 100%;
text-align: center;
}
#dataTable td, #dataTable th {
border: 1px solid #090902;
padding: 8px;
}
#dataTable tr:nth-child(even){background-color: #e2e1e1;}
#dataTable tr:hover {background-color: #ffff4d;}
#dataTable th {
padding-top: 12px;
padding-bottom: 12px;
text-align: center;
background-color: #ffc04d;
color: white;
}
</style>
</head>
<body>
<div>
<table id="dataTable">
<tr><th>Time</th><th>Log</th></tr>
</table>
</div>
<br>
<br>
<script>
var Avalues = [];
//var timeStamp = [];
var dateStamp = [];
setInterval(function() {
// Call a function repetatively with 5 Second interval
getData();
}, 3000); //5000mSeconds update rate
function getData() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
//Push the data in array
// var time = new Date().toLocaleTimeString();
var date = new Date();
var txt = this.responseText;
var obj = JSON.parse(txt);
Avalues.push(obj.Log);
// timeStamp.push(time);
dateStamp.push(date);
//Update Data Table
var table = document.getElementById("dataTable");
var row = table.insertRow(1); //Add after headings
var cell1 = row.insertCell(0);
var cell2 = row.insertCell(1);
cell1.innerHTML = date;
//cell2.innerHTML = time;
cell2.innerHTML = obj.Log;
}
};
xhttp.open("GET", "readData", true); //Handle readData server on ESP8266
xhttp.send();
}
</script>
</body>
</html>
)=====";
void handleRoot() {
String s = MAIN_page; //Read HTML contents
server.send(200, "text/html", s); //Send web page
}
void readData() {
lcd.init(); // initialize the lcd
// Print a message to the LCD.
lcd.backlight();
int state = digitalRead(MetalTouch); //Continuously check the state of Metal sensor
delay(500); //Check state of Metal after every half second
lcd.setCursor(0,0);
lcd.print("Metal Body Scan");
lcd.setCursor(0,1);
lcd.print("....No Touch....");
Serial.print(state);
if(state == HIGH){
digitalWrite (buzzer, HIGH); //If intrusion detected ring the buzzer
lcd.setCursor(0,1);
lcd.print(" Body Touched ");
delay(1000);
digitalWrite (buzzer, LOW);
lcd.setCursor(0,1);
lcd.print("Sent to HTTP >>>");
delay(1000);
Message = "Metal Body Touched";
String data = "{\"Log\":\""+ String(Message) +"\"}";
server.send(200, "text/plane", data); //Send ADC value, temperature and humidity JSON to client ajax request
Serial.println("Metal Body Touched");
}
}
void setup() {
Serial.begin(9600);
lcd.init(); // initialize the lcd
// Print a message to the LCD.
lcd.backlight();
Serial.print("Connecting to Wifi Network");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("Successfully connected to WiFi.");
Serial.println("IP address is : ");
Serial.println(WiFi.localIP());
lcd.setCursor(0,0);
lcd.print(WiFi.localIP());
delay(2000);
lcd.clear();
server.on("/", handleRoot); //Which routine to handle at root location. This is display page
server.on("/readData", readData); //This page is called by java Script AJAX
server.begin(); //Start server
Serial.println("HTTP server started");
pinMode(MetalTouch, INPUT); // Metal sensor as input
pinMode(buzzer, OUTPUT); // Buzzer alaram as output
digitalWrite (buzzer, LOW);// Initially buzzer off
}
void loop(){
server.handleClient(); //Handle client requests
}
Then, upload the code to your NodeMCU board. Make sure you have selected the right board and COM port. Also, make sure you’ve inserted your WiFi Credentials in the code.
After a successful upload, open the Serial Monitor at a baud rate of 9600. Press the “EN/RST” button on the ESP8266 board. Now it should print its IP address. After That Open web address and Type IP address in address bar and get result.